Wanting to become a Security Auditor? Learn by doing.
Using the content from previous Secureum RACE epochs, you can learn by doing and in the same way as they intended. Made by Security Researchers for Security Researchers.
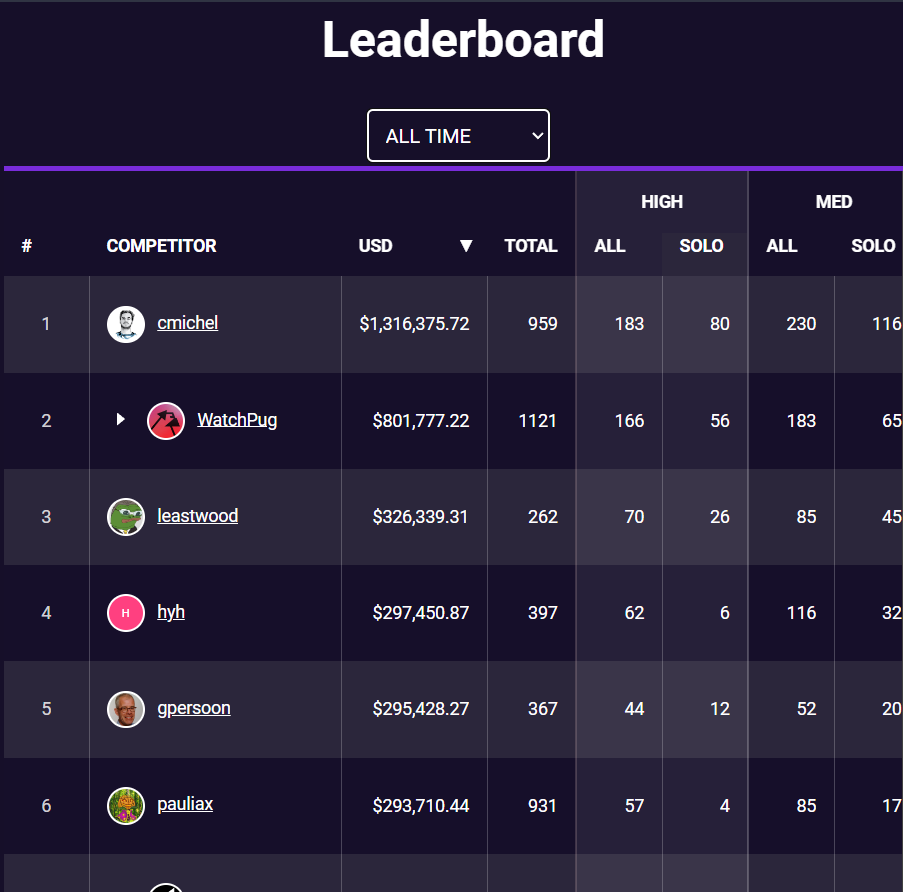
pragma solidity 0.8.10;
import "@openzeppelin/.../token/ERC20/IERC20.sol";
import "@openzeppelin/.../token/ERC20/extensions/IERC20Metadata.sol";
import "@openzeppelin/.../utils/Context.sol";
contract InSecureum is Context, IERC20, IERC20Metadata {
mapping(address => uint256) private _balances;
mapping(address => mapping(address => uint256)) private _allowances;
uint256 private _totalSupply;
string private _name;
string private _symbol;
constructor(string memory name_, string memory symbol_) {
_name = name_;
_symbol = symbol_;
}
function name() public view virtual override returns (string memory) {
return _name;
}
function symbol() public view virtual override returns (string memory) {
return _symbol;
}
function decimals() public view virtual override returns (uint8) {
return 8;
}
function totalSupply() public view virtual override returns (uint256) {
return _totalSupply;
}
function balanceOf(address account)
public
view
virtual
override
returns (uint256)
{
return _balances[account];
}
function transfer(address recipient, uint256 amount)
public
virtual
override
returns (bool)
{
_transfer(_msgSender(), recipient, amount);
return true;
}
function allowance(address owner, address spender)
public
view
virtual
override
returns (uint256)
{
return _allowances[owner][spender];
}
function approve(address spender, uint256 amount)
public
virtual
override
returns (bool)
{
_approve(_msgSender(), spender, amount);
return true;
}
function transferFrom(
address sender,
address recipient,
uint256 amount
) public virtual override returns (bool) {
uint256 currentAllowance = _allowances[_msgSender()][sender];
if (currentAllowance != type(uint256).max) {
unchecked {
_approve(sender, _msgSender(), currentAllowance - amount);
}
}
_transfer(sender, recipient, amount);
return true;
}
function increaseAllowance(address spender, uint256 addedValue)
public
virtual
returns (bool)
{
_approve(
_msgSender(),
spender,
_allowances[_msgSender()][spender] + addedValue
);
return true;
}
function decreaseAllowance(address spender, uint256 subtractedValue)
public
virtual
returns (bool)
{
uint256 currentAllowance = _allowances[_msgSender()][spender];
require(
currentAllowance > subtractedValue,
"ERC20: decreased allowance below zero"
);
_approve(_msgSender(), spender, currentAllowance - subtractedValue);
return true;
}
function _transfer(
address sender,
address recipient,
uint256 amount
) internal virtual {
require(sender != address(0), "ERC20: transfer from the zero address");
require(recipient != address(0), "ERC20: transfer to the zero address");
uint256 senderBalance = _balances[sender];
require(
senderBalance >= amount,
"ERC20: transfer amount exceeds balance"
);
unchecked {
_balances[sender] = senderBalance - amount;
}
_balances[recipient] += amount;
emit Transfer(sender, recipient, amount);
}
function _mint(address account, uint256 amount) external virtual {
_totalSupply += amount;
_balances[account] = amount;
emit Transfer(address(0), account, amount);
}
function _burn(address account, uint256 amount) internal virtual {
require(account != address(0), "ERC20: burn from the zero address");
require(
_balances[account] >= amount,
"ERC20: burn amount exceeds balance"
);
unchecked {
_balances[account] = _balances[account] - amount;
}
_totalSupply -= amount;
emit Transfer(address(0), account, amount);
}
function _approve(
address owner,
address spender,
uint256 amount
) internal virtual {
require(spender != address(0), "ERC20: approve from the zero address");
require(owner != address(0), "ERC20: approve to the zero address");
_allowances[owner][spender] += amount;
emit Approval(owner, spender, amount);
}
}
Inspired by companies like:
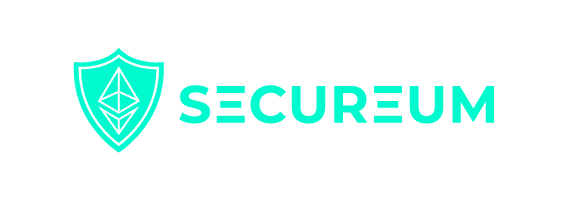
Goal setting for succesfully auditing in contests like these?
Get some monetary motivation of popular security research platforms such as
Immunefi
$10,000,000
MakerDAO
Immunefi
$5,000,000
GMX
Immunefi
$3,333,333
OlympusDAO
Code4rena
$30,0000
Blockswap
Code4rena
$36,500
RabbitHole Quest
Code4rena
$90,500
Drips Protocol
Sherlock
$720,000
Optimism
Sherlock
$70,000
Derby
Sherlock
$72,500
UXD Protocol
Learn by doing!
The quizzes that you'll take are taken from the each Secureum Race epoch.
You can complete them over and over, with or without time limits!
But they won't complete themselves.
Some things you might want to know
Frequently asked questions
Are we ranked or graded?
Yes, you will receive your grade once you complete the race.
Does this count towards anything?
Not anything official, but hopefully, it can help you improve your security posture!
Will this teach me how to become a top security researcher?
It will help you to benchmark your own learning and growth as a researcher. It will also hopefully teach you a few new things along the way.
Why should I complete past races?
Each race is a unique and interesting puzzle. Completing them may help you learn new skills or review old skills in a different way.
What do I do once I complete all of the races?
You can write a blog post about your experience and share it with the community!